What Makes an LLM an Agent? Understanding the Evolution from Language Models to AI Agents
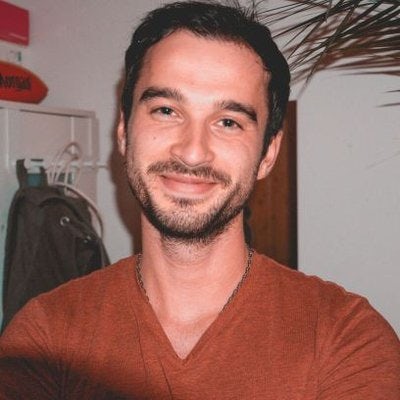
- Published on
The Question That Started It All
Recently, I found myself pondering a fundamental question that many developers in the AI space encounter: What exactly is the difference between a Large Language Model (LLM) and an AI Agent? During my research journey, I discovered that the answer lies in a crucial distinction - autonomy and decision-making capabilities.
The Key Difference: More Than Just Responses
While LLMs are impressive in their ability to process and generate text, they essentially operate as sophisticated response generators. They take in prompts and produce outputs based on their training. An AI Agent, however, takes this several steps further by implementing what we call the ReACT pattern:
- Reasoning: Analyzing the situation and planning actions
- Action: Executing specific tools or functions
- Observation: Processing the results and deciding next steps
Building a Simple AI Agent
To demonstrate this concept, let's build a simple arithmetic AI Agent using Langgraph. This example will show how an agent can perform calculations by utilizing tools and making decisions.
The Complete Code Overview
First, let's look at our complete implementation:

Breaking Down the Components
1. Tool Definition
We start by defining our mathematical tools that the agent can use:
tools = [add, multiply, divide]
llm = ChatOpenAI(model="gpt-4", api_key="sk-")
llm_with_tools = llm.bind_tools(tools)
2. System Configuration
We set up the agent's role and capabilities:
sys_msg = SystemMessage(content="You are a helpful assistant tasked with performing arithmetic on a set of inputs.")
3. Assistant Node
The core decision-making component:
def assistant(state: MessagesState):
return {"messages": [llm_with_tools.invoke([sys_msg] + state["messages"])]}
4. Graph Construction - Nodes
Here's where define our nodes, nodes are the steps in our application so basically the "work units":
builder = StateGraph(MessagesState)
builder.add_node("assistant", assistant)
builder.add_node("tools", ToolNode(tools))
4. Graph Construction - Edges
The most crucial part of our implementation is how we connect these units. We use edges for simple routing and conditional_edges where we can define logic that determines where to route next.
builder.add_edge(START, "assistant")
builder.add_conditional_edges("assistant", tools_condition)
builder.add_edge("tools", "assistant")
This last line builder.add_edge("tools", "assistant")
is particularly important - it creates the feedback loop that makes our LLM truly an agent. After a tool executes, the result flows back to the assistant, allowing it to:
- Evaluate the result
- Decide if more actions are needed
- Plan the next step
Our Agent in action:
When we run our agent with a complex arithmetic query:
messages = [HumanMessage(content="Add 3 and 4. Multiply the output by 2. Divide the output by 5")]
messages = react_graph.invoke({"messages": messages})
The agent follows this process:
- Understands the multi-step arithmetic problem
- Calls the add function with 3 and 4
- Receives the result (7)
- Calls multiply with 7 and 2
- Receives the result (14)
- Finally calls divide with 14 and 5
- Returns the final result (2.8)

Conclusion
The evolution from LLMs to AI Agents represents a significant leap in artificial intelligence. While LLMs excel at understanding and generating text, AI Agents take this a step further by adding autonomous decision-making and action-taking capabilities. As we continue to develop these technologies, the distinction between "knowing" and "doing" becomes increasingly important in our AI systems.