Difference between AWS CDK Construct, Stack, App
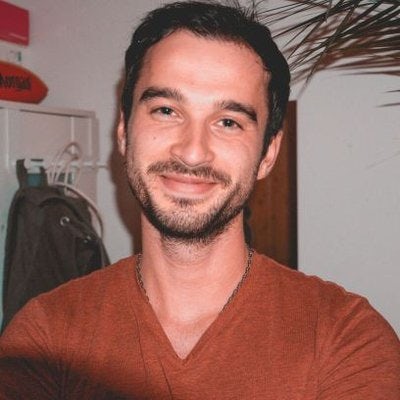
- Published on
While developing in AWS CDK these terms (Stack, Construct, App) come often along. I wanted to get a better understanding, so I did a little reasearch about the differences. I made a summary for you, so you can get the core idea behind these terms easily.
In AWS CDK, there are three key concepts: Constructs, Stacks, and App. Here's a breakdown of each:
(image of relationship between construct, stack, app in aws CDK)
- Constructs: ◦ Constructs are the basic building blocks of AWS CDK apps. They represent cloud components and encapsulate everything AWS CloudFormation needs to create the component. ◦ Constructs can represent a single AWS resource, such as an S3 bucket, or a higher-level abstraction consisting of multiple related AWS resources. ◦ The AWS CDK includes a collection of constructs called the AWS Construct Library, which contains constructs for every AWS service. Construct Hub: https://constructs.dev/search?q=&cdk=aws-cdk&cdkver=2&sort=downloadsDesc&offset=0
// 👇 create the EC2 instance
const ec2Instance = new ec2.Instance(this, 'ec2-instance', {
vpc,
vpcSubnets: {
subnetType: ec2.SubnetType.PUBLIC,
},
securityGroup: ec2InstanceSG,
instanceType: ec2.InstanceType.of(
ec2.InstanceClass.BURSTABLE2,
ec2.InstanceSize.MICRO,
),
machineImage: new ec2.AmazonLinuxImage({
generation: ec2.AmazonLinuxGeneration.AMAZON_LINUX_2,
}),
keyName: 'ec2-key-pair',
});
}
- Stacks: ◦ Stacks are equivalent to CloudFormation stacks and are made up of Constructs. ◦ A Stack represents a CF template in CDK terms, while a Construct represents the AWS resources you want to create. ◦ Stacks are deployed as a unit and can contain multiple Constructs.
export class CdkStarterStack extends cdk.Stack {
constructor(scope: cdk.App, id: string, props?: cdk.StackProps) {
super(scope, id, props);
// ... rest
// 👇 create RDS instance
const dbInstance = new rds.DatabaseInstance(this, 'db-instance', {
vpc,
vpcSubnets: {
subnetType: ec2.SubnetType.PRIVATE_ISOLATED,
},
engine: rds.DatabaseInstanceEngine.postgres({
version: rds.PostgresEngineVersion.VER_13_1,
}),
instanceType: ec2.InstanceType.of(
ec2.InstanceClass.BURSTABLE3,
ec2.InstanceSize.MICRO,
),
credentials: rds.Cre
......
You create a stack to group constructs that work and belong together. For example you create the Website Stack that contains the S3 Construct (storing the files), a Route53 (for domain handling) Construct and also a CloudFront Construct (for CDN stuff). As you can see all these 3 constructs have something in common to setup a website in AWS, so we group them in a stack.
- Apps: ◦ Apps are the root objects in AWS CDK and contain Stacks. ◦ An App is the starting point of a CDK project and serves as the root of the Construct tree.
import * as cdk from 'aws-cdk-lib';
import {CdkStarterStack} from '../lib/cdk-starter-stack';
const app = new cdk.App();
new CdkStarterStack(app, 'cdk-stack', {
stackName: 'cdk-stack',
env: {
region: process.env.CDK_DEFAULT_REGION,
account: process.env.CDK_DEFAULT_ACCOUNT,
},
});