Nextjs 13 - New API structure
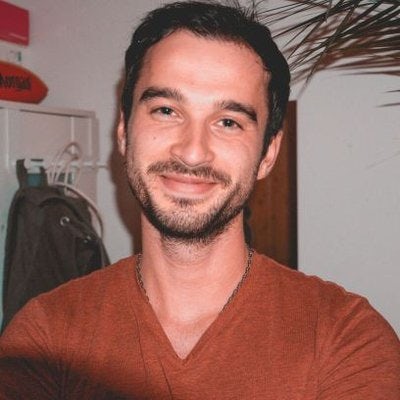
- Published on
Next.js 13 - New API structure
The new app directory for Next.js just got even better. To define an API route, all you need to do is create a file named "route.tsx" in the app directory. Once you've created the file, you can start defining your API endpoint. However, it's important to note that API routes now use the standard Request object, rather than the express-like req and res objects.
When defining an API route, you export the handlers for the methods you want to support. For example, if you want to support both the GET and POST methods, you can export both the GET and POST functions.
Here's an example code snippet:
import { NextResponse } from 'next/server';
export async function GET() {
return NextResponse.json({ hello: 'world' });
}
export async function POST(request: Request) {
const body = await request.json();
const data = await getData(body);
return NextResponse.json(data);
}
As you can see, the GET function simply returns a JSON response with the "hello" key and value. The POST function is a bit more complex as it takes in the Request object and parses the body of the request to retrieve the data. It then returns a JSON response with the retrieved data.
If you want to manipulate the response, you can use the NextResponse object. For example, if you want to set cookies, you can do so like this:
export async function POST(request: Request) {
const organizationId = getOrganizationId(resizeTo());
const response = NextResponse.json({ organizationId });
response.cookie('organizationId', organizationId);
return response;
}
In this example, we're setting a cookie with the key "organizationId" and the value of the retrieved organization ID. This can be incredibly useful for authentication purposes or remembering user preferences.
In conclusion, the new app directory for Next.js now supports API routes, making it easier than ever to create custom backend routes for your applications. With the ability to define handlers for multiple HTTP methods and manipulate responses with the NextResponse object, developers have a lot of flexibility and power at their fingertips. So why not give it a try and create your own API endpoint today?
If you found this content helpful ⇢